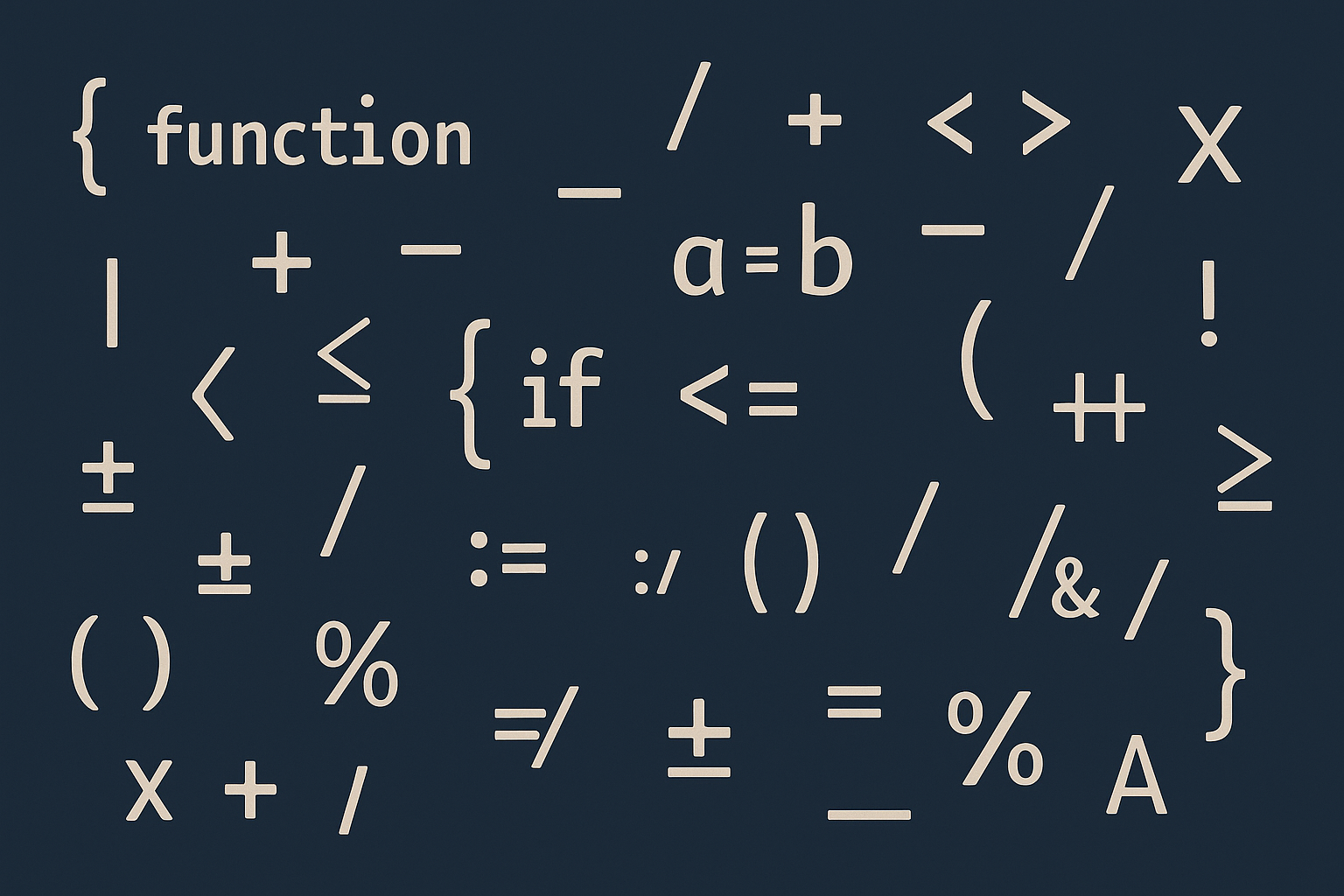
10 Coding Interview Questions Every Developer Should Practice
Table of Contents
- The Real Test Behind Every Coding Interview
- The Process That Never Fails
- Here are 10 questions every developer should deeply reflect on
- 1. How Do You Reverse a Linked List?
- 2. Can You Find the First Non-Repeating Character in a String?
- 3. How Would You Merge Two Sorted Arrays?
- 4. How Do You Detect a Cycle in a Linked List?
- 5. How Would You Generate All Subsets of a Set?
- 6. Can You Rotate a Matrix by 90 Degrees?
- 7. How Do You Find the Missing Number in a Range?
- 8. Can You Implement a Queue Using Two Stacks?
- 9. How Would You Validate a Binary Search Tree?
- 10. Can You Find the Longest Substring Without Repeating Characters?
- Final Thoughts: Practice Thinking, Not Just Coding
The Real Test Behind Every Coding Interview
After spending over a decade in tech, building systems, mentoring engineers, and conducting countless interviews, one thing is clear: coding interviews are less about code, and more about clarity of thought. The keyboard is not your weapon; your mind is. Syntax can be learned, but the ability to dissect a problem, hold multiple possibilities in your head, and communicate a clear path forward, that’s what differentiates strong engineers from average ones.
In an interview setting, you're asked to build something in minutes that, in real life, might take hours or days. It’s not a fair test, but it’s a revealing one. It tests your fundamentals, how well you understand trade-offs, how quickly you can simplify a problem, and whether you crumble under ambiguity or stay calm and ask the right questions.
The Process That Never Fails
When you’re handed a coding question, don’t start coding. Pause. Ask clarifying questions. Make sure you’ve understood the problem deeply, the inputs, the outputs, the constraints. Then, before thinking in syntax, think in structure. Walk through the logic out loud. Write pseudocode. When that looks sound, only then write actual code. This habit doesn’t just help in interviews, it scales to real-world engineering.
Here are 10 questions every developer should deeply reflect on
1. How Do You Reverse a Linked List?
It’s deceptively simple. But what it actually tests is your understanding of pointers, memory, and iterative logic. Most candidates get stuck not on logic, but on pointer handling. If you can explain what’s happening to each node clearly, you’ve already impressed the interviewer.
2. Can You Find the First Non-Repeating Character in a String?
This question looks like a beginner-level string manipulation problem, but it reveals how you approach frequency counting, use hash maps, and whether you naturally think in terms of time-space complexity.
3. How Would You Merge Two Sorted Arrays?
Sorting is easy when you rely on built-ins. But merging manually tests your ability to maintain invariants, handle edge cases, and preserve sorted order without unnecessary memory waste. A classic problem to assess clean logic.
4. How Do You Detect a Cycle in a Linked List?
This is where theoretical knowledge meets practical insight. Floyd’s Cycle Detection isn’t just an algorithm, it’s a way to check if you understand how slow/fast pointer mechanics help solve complex problems efficiently.
5. How Would You Generate All Subsets of a Set?
This dives into recursion and backtracking. It tests whether you understand how to think recursively, visualize the recursion tree, and manage branching decisions. One of the purest ways to evaluate logical depth.
6. Can You Rotate a Matrix by 90 Degrees?
Matrix rotation is spatial. It requires you to understand index mapping and perform in-place transformations. What the interviewer is really looking for is your ability to reason through multi-dimensional data and loop management.
7. How Do You Find the Missing Number in a Range?
This number theory puzzle checks whether you can move from brute-force to elegant mathematical solutions using formulas. It also subtly evaluates your handling of off-by-one and indexing errors, common bugs in production code.
8. Can You Implement a Queue Using Two Stacks?
Simulating one data structure with another shows how well you’ve internalized stack and queue behavior. It’s not about cleverness, it’s about knowing how fundamental tools work, and how they can emulate each other through operation inversion.
9. How Would You Validate a Binary Search Tree?
This is where recursion meets correctness. Interviewers look to see if you understand the global ordering constraint of a BST, not just local comparisons. It reveals how well you track state across recursive calls.
10. Can You Find the Longest Substring Without Repeating Characters?
This is the classic sliding window problem. It tests your ability to optimize a brute-force solution into linear time using efficient state tracking. It's especially valuable because the sliding window approach appears in many advanced problems too.
Final Thoughts: Practice Thinking, Not Just Coding
These aren’t just problems, they’re mirrors. Each one reflects how you think, how you approach structure, and how you manage ambiguity. Practice them not to memorize solutions, but to train your mind to recognize patterns and adapt logic under pressure.
If you want to simulate real interviews with voice-first AI, try Groco at groco.codeaetheris.com. It forces you to speak your logic , just like in real interviews, so you can build clarity and confidence that lasts.